Does SIMD Improve the Performance of Node.js?
Single instruction, multiple data (SIMD) instructions, such as AVX on x64 processors, are designed to improve performance. In some areas, such as numerical computation, they offer significant performance gains. In some other areas, they provide less gain or even performance loss. Do they improve the performance of Node.js? We ran some experiments. ...
Add the Current Copyright Year to the HTML File in a Vite Project
In a project built by Vite, index.html is usually the entry point of the project. While we can put the copyright notice in the JavaScript bundle, it is often desirable to add it directly to the HTML file for various reasons. For example, maybe the copyright notice is entangled with the privacy policy or terms of service links, which we usually want to make visible even when the user turns off JavaScript. A straightforward solution is to add a copyright notice with a fixed year to the footer like: <footer> Copyright © 2024 My Name </footer> However, it can be quite annoying to bump the year in every project, even though it’s only once per year. In this post, we present a simple and clean solution to address this problem using the HTML transformation hook of Vite. This solution does not use in-page JavaScript so that users can see them even without JavaScript enabled. We have also created a package @8hobbies/vite-plugin-year that uses the solution from this post for your convenience. ...
Guides to Promoting a JavaScript Open Source Project
You just have created a new JavaScript open source project. Now you wonder, how do I promote it? Having been devoted to open source for over a decade, I’d like to share how I usually approach it. Many of these ideas apply to not only JavaScript projects but also open source software projects in general. There are 4 aspects to focus on: Value Addition High Code Quality More Exposure to Potential Users Lower Barrier to Use the Project ...
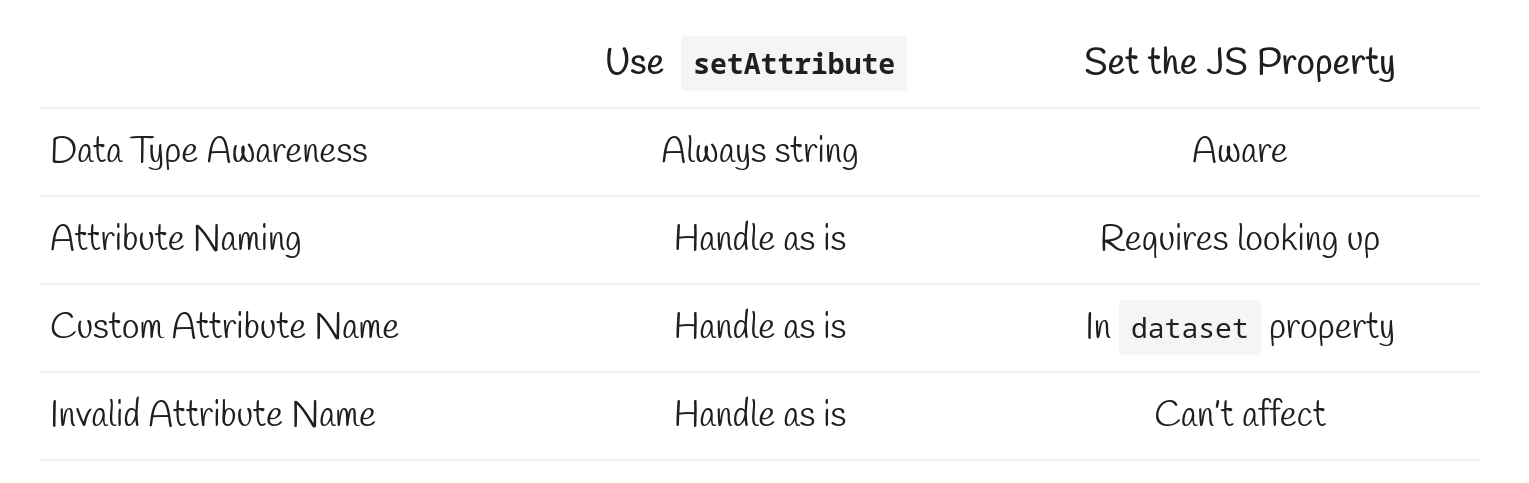
Add/Update/Remove an HTML Attribute in JavaScript
HTML attributes are name-value pairs, separated by =, in the start tag of an element after the element name, such as id=blog-link and href="https://8hob.io" below: <a id="blog-link" href="https://8hob.io">8 Hobbies JavaScript Blog</a> How do we add, update, and remove an HTML attribute in JavaScript (JS)? ...
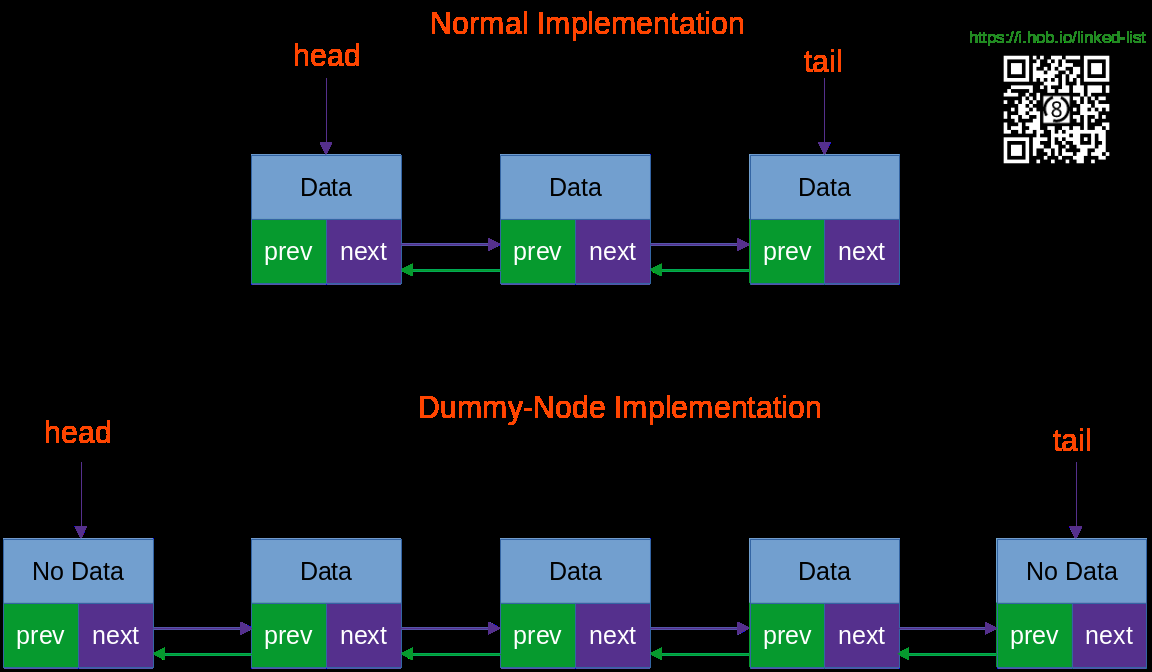
Simple and Error-Free Linked List Implementation With a Dummy Head/Tail Node
Linked lists are fundamental data structures frequently used in computer science. Often, programmers need to directly implement them. How can we implement an error-free linked list quickly, especially under time pressure and mental stress during job interviews? This post discusses an important trick: Using a dummy head/tail node. ...